![]() |
悲伤的山羊 · 蓝牙版T8FB常见问题· 5 月前 · |
![]() |
聪明伶俐的电影票 · 新站、新线“首秀” ...· 6 月前 · |
![]() |
爱看书的盒饭 · 提森-博内米萨博物馆 - 每日环球展览 - ...· 6 月前 · |
![]() |
逆袭的大象 · 计算机学院在全国大学生计算机博弈大赛成绩优秀 ...· 6 月前 · |
![]() |
私奔的数据线 · 香江廿年⑲|香港青少年制服团体:一个公民教育 ...· 7 月前 · |
ViewPager is a layout manager that allows the user to flip left and right through pages of data. It is mostly found in apps like Youtube, Snapchat where the user shifts right – left to switch to a screen. Instead of using activities fragments are used. It is also used to guide the user through the app when the user launches the app for the first time.
Steps for implementing viewpager:
An adapter populates the pages inside the Viewpager. PagerAdapter is the base class which is extended by FragmentPagerAdapter and FragmentStatePagerAdapter. Let’s see a quick difference between the two classes.
Difference between FragmentPagerAdapter and FragmentStatePagerAdapter:
Following is the structure of the ViewPagerAdapter Class:
import androidx.annotation.NonNull;
import androidx.annotation.Nullable;
import androidx.fragment.app.Fragment;
import androidx.fragment.app.FragmentManager;
import androidx.fragment.app.FragmentPagerAdapter;
import java.util.ArrayList;
import java.util.List;
public class ViewPagerAdapter extends FragmentPagerAdapter {
private final List<Fragment> fragments = new ArrayList<>();
private final List<String> fragmentTitle = new ArrayList<>();
public ViewPagerAdapter(@NonNull FragmentManager fm)
super(fm);
public void add(Fragment fragment, String title)
fragments.add(fragment);
fragmentTitle.add(title);
@NonNull @Override public Fragment getItem(int position)
return fragments.get(position);
@Override public int getCount()
return fragments.size();
@Nullable
@Override
public CharSequence getPageTitle(int position)
return fragmentTitle.get(position);
Method Description:
- getCount(): This method returns the number of fragments to display. (Required to Override)
- getItem(int pos): Returns the fragment at the pos index. (Required to override)
- ViewPagerAdapter(@NonNull FragmentManager FM): (required) The ViewPager Adapter needs to have a parameterized constructor that accepts the FragmentManager instance. Which is responsible for managing the fragments. A FragmentManager manages Fragments in Android, specifically, it handles transactions between fragments. A transaction is a way to add, replace, or remove fragments.
- getPageTitle(int pos): (optional) Similar to getItem() this methods returns the title of the page at index pos.
- add(Fragment fragment, String title): This method is responsible for populating the fragments and fragmentTitle lists. which hold the fragments and titles respectively.
Example
A sample GIF is given below to get an idea about what we are going to do in this article. Note that we are going to implement this project using the Java language.
Step by Step Implementation
Step 1: Create a New Project
To create a new project in Android Studio please refer to How to Create/Start a New Project in Android Studio. Note that select Java as the programming language. Initially, the project directory should look like this.
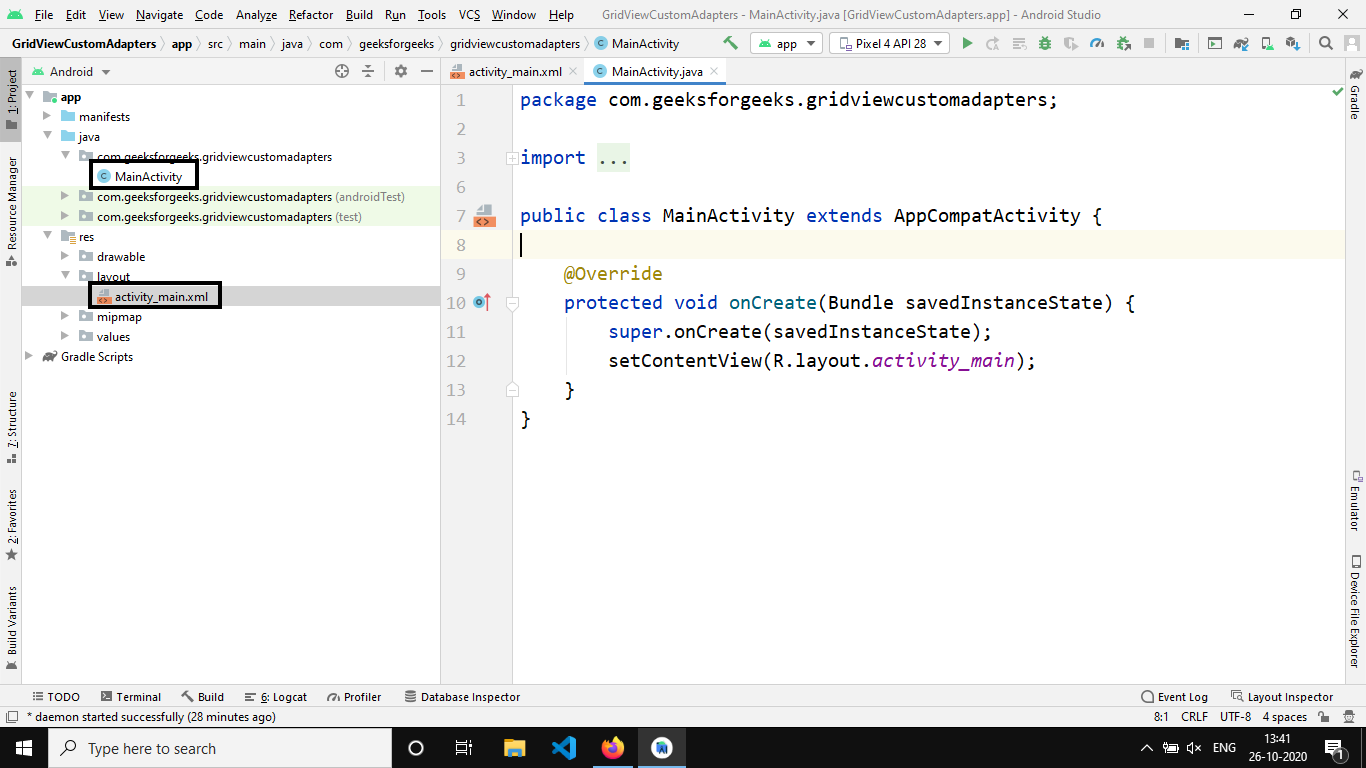
Step 2: Working with the activity_main.xml file
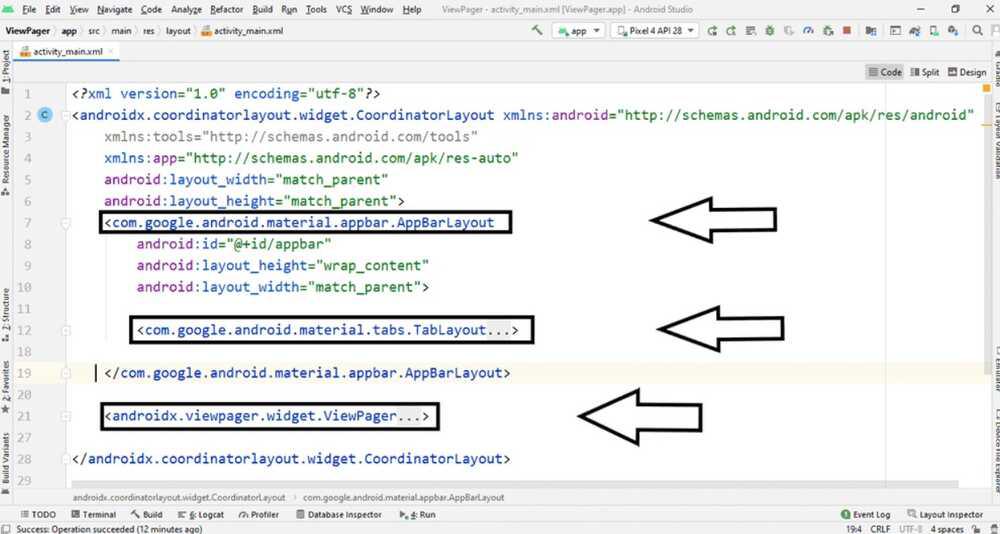
The three widgets AppBarLayout used to host the TabLayout which is responsible for displaying the page titles. ViewPager layout which will house the different fragments. The below Image explains the important parameters to set to get the app working as intended.
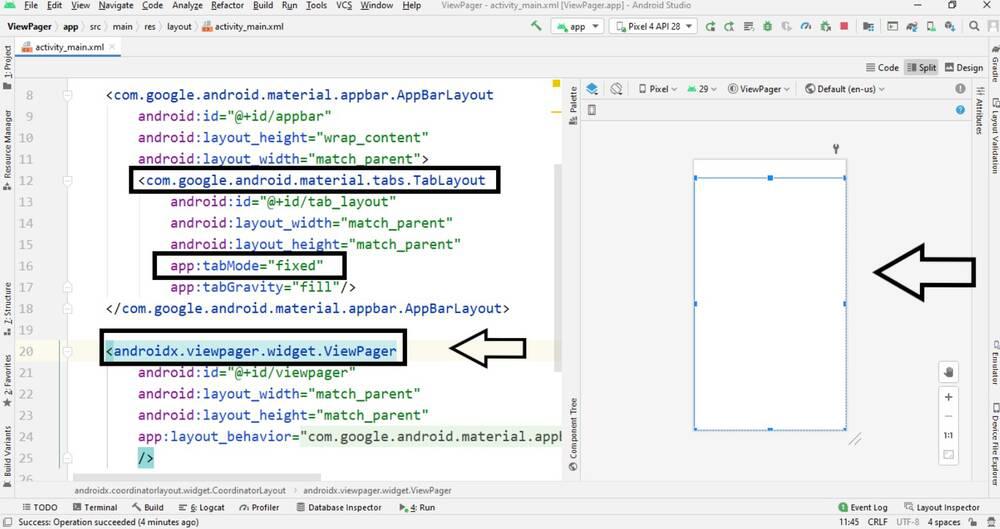
In the TabLayout we need to add the tabmode = “fixed” parameter which tells the android system that will have a fixed number of tabs in our application. Add the following code to the “activity_main.xml” file.
<?xml version="1.0" encoding="utf-8"?>
<androidx.coordinatorlayout.widget.CoordinatorLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent">
<com.google.android.material.appbar.AppBarLayout
android:id="@+id/appbar"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<com.google.android.material.tabs.TabLayout
android:id="@+id/tab_layout"
android:layout_width="match_parent"
android:layout_height="match_parent"
app:tabGravity="fill"
app:tabMode="fixed" />
</com.google.android.material.appbar.AppBarLayout>
<androidx.viewpager.widget.ViewPager
android:id="@+id/viewpager"
android:layout_width="match_parent"
android:layout_height="match_parent"
app:layout_behavior="@string/appbar_scrolling_view_behavior" />
</androidx.coordinatorlayout.widget.CoordinatorLayout>
Step 3: Create Fragments
Now we need to design out pages that are fragmented. For this tutorial, we will be using three Pages (fragments). Add three blank fragments to the project. The project Structure should look like this.
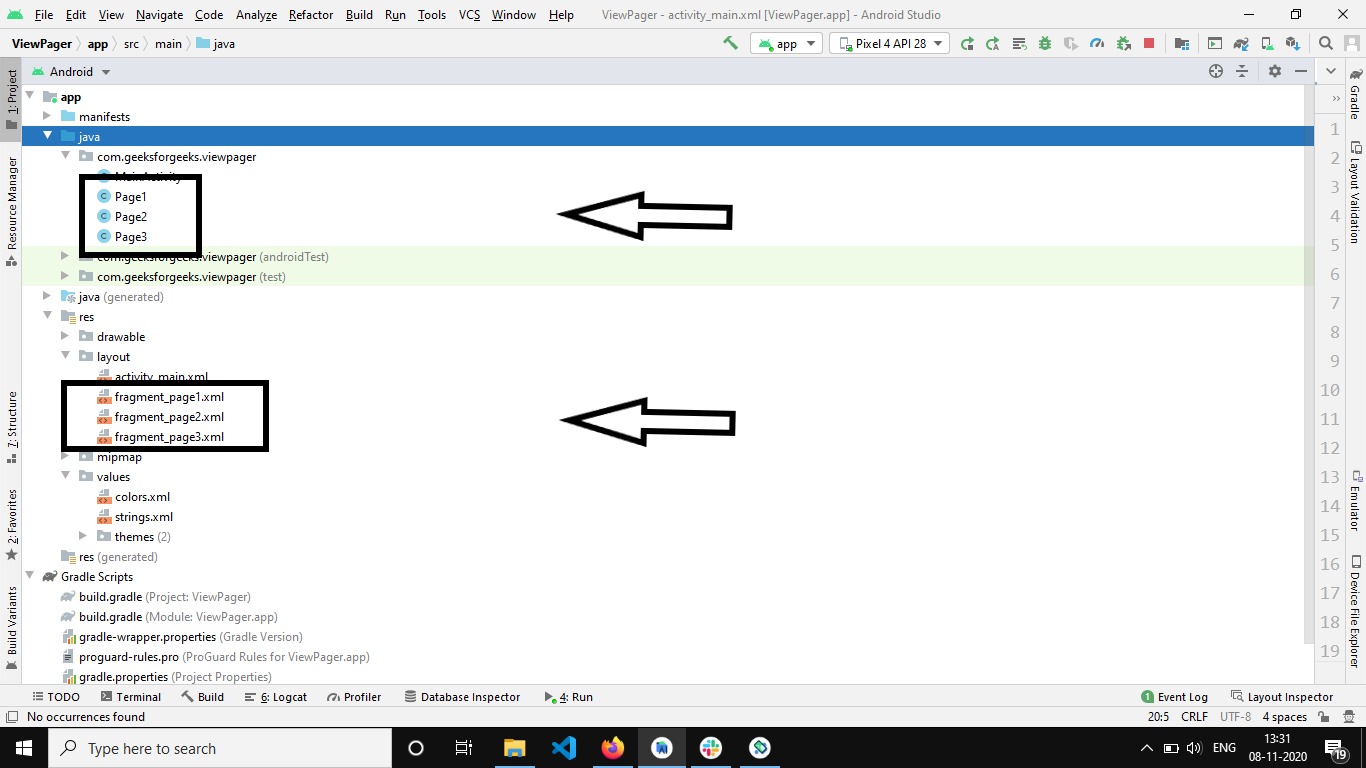
Below is the code for the Page1.java, Page2.java, and Page3.java file respectively.
import android.os.Bundle;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import androidx.annotation.NonNull;
import androidx.annotation.Nullable;
import androidx.fragment.app.Fragment;
public class Page1 extends Fragment {
public Page1() {
// required empty public constructor.
@Override
public void onCreate(@Nullable Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
@Nullable
@Override
public View onCreateView(@NonNull LayoutInflater inflater, @Nullable ViewGroup
container, @Nullable Bundle savedInstanceState) {
return inflater.inflate(R.layout.fragment_page1, container, false);
import android.os.Bundle;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import androidx.annotation.NonNull;
import androidx.annotation.Nullable;
import androidx.fragment.app.Fragment;
public class Page2 extends Fragment {
public Page2() {
// required empty public constructor.
@Override
public void onCreate(@Nullable Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
@Nullable
@Override
public View onCreateView(@NonNull LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) {
return inflater.inflate(R.layout.fragment_page2, container, false);
import android.os.Bundle;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import androidx.annotation.NonNull;
import androidx.annotation.Nullable;
import androidx.fragment.app.Fragment;
public class Page3 extends Fragment {
public Page3() {
// required empty public constructor.
@Override
public void onCreate(@Nullable Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
@Nullable
@Override
public View onCreateView(@NonNull LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) {
return inflater.inflate(R.layout.fragment_page3, container, false);
Method Description:
- Page1(): Default Constructure.
- onCreateView( onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState): This method is responsible for inflating (parsing) the respective XML file and return the view which is added to the ViewPager adapter.
- onCreate(Bundle SaveInstanceState): This methods is similar to activities OnCreate() method.
Designing the Page XML Files. All the fragments XML layouts have the same designs. We have a TextView at the center displaying the name of the respective page, the root container used here is FrameLayout whose background is set to #0F9D58
Below is the code for the fragment_page1.xml file:
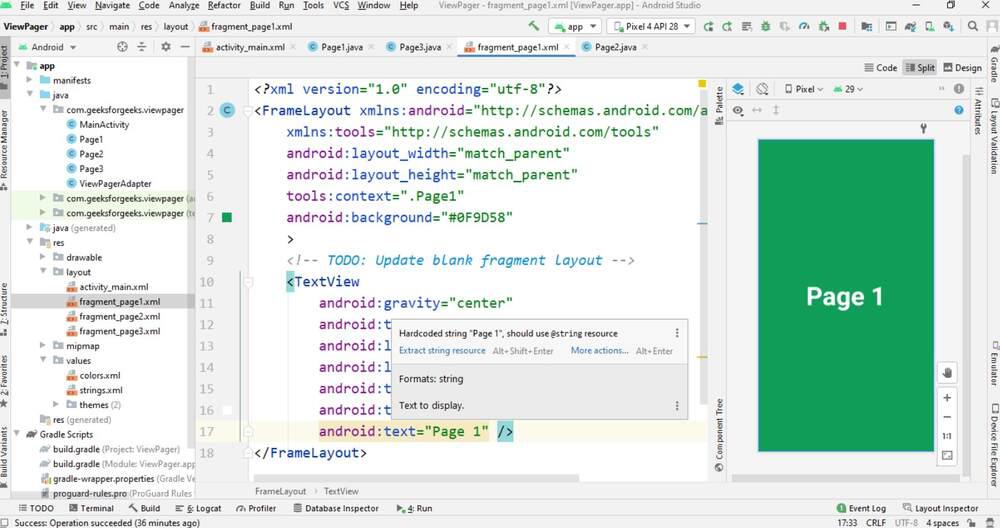
Code:
<?xml version="1.0" encoding="utf-8"?>
<FrameLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#0F9D58"
tools:context=".Page1">
<!-- TODO: Update blank fragment layout -->
<TextView
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center"
android:text="Page 1"
android:textColor="@color/white"
android:textSize="60sp"
android:textStyle="bold" />
</FrameLayout>
Below is the code for the fragment_page2.xml file:
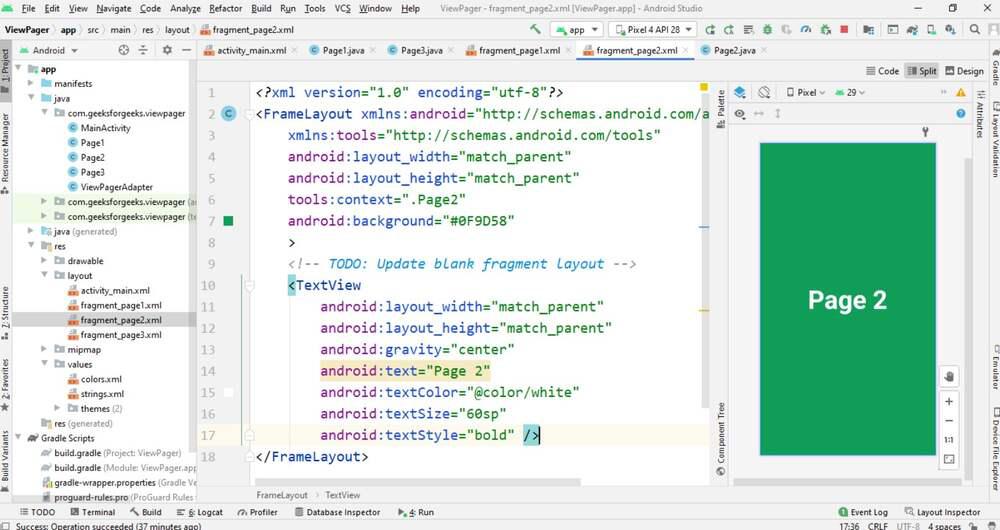
Code:
<?xml version="1.0" encoding="utf-8"?>
<FrameLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#0F9D58"
tools:context=".Page2">
<!-- TODO: Update blank fragment layout -->
<TextView
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center"
android:text="Page 2"
android:textColor="@color/white"
android:textSize="60sp"
android:textStyle="bold" />
</FrameLayout>
Below is the code for the fragment_page3.xml file:
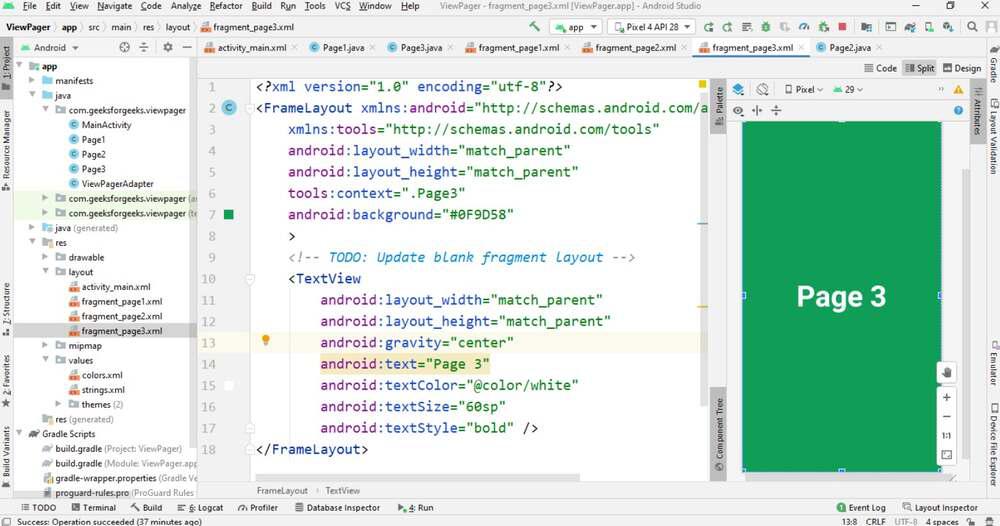
Code:
<?xml version="1.0" encoding="utf-8"?>
<FrameLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#0F9D58"
tools:context=".Page3">
<!-- TODO: Update blank fragment layout -->
<TextView
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center"
android:text="Page 3"
android:textColor="@color/white"
android:textSize="60sp"
android:textStyle="bold" />
</FrameLayout>
Step 4: Creating the ViewPager Adapter
Add a java class named ViewPagerAdapter to the project structure. The project structure would look like this.
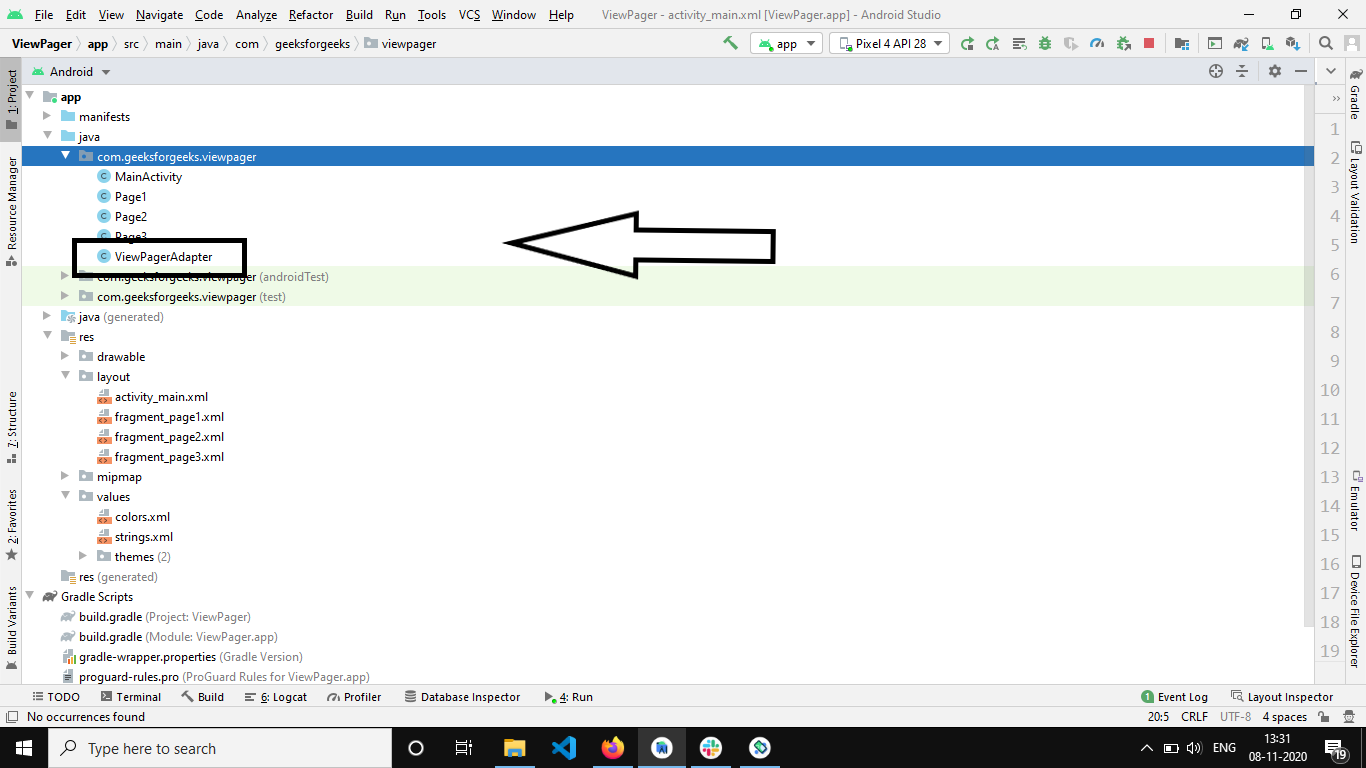
Below is the code for the ViewPagerAdapter.java file:
import androidx.annotation.NonNull;
import androidx.annotation.Nullable;
import androidx.fragment.app.Fragment;
import androidx.fragment.app.FragmentManager;
import androidx.fragment.app.FragmentPagerAdapter;
import
java.util.ArrayList;
import java.util.List;
public class ViewPagerAdapter extends FragmentPagerAdapter {
private final List<Fragment> fragments = new ArrayList<>();
private final List<String> fragmentTitle = new ArrayList<>();
public ViewPagerAdapter(@NonNull FragmentManager fm) {
super(fm);
public void add(Fragment fragment, String title) {
fragments.add(fragment);
fragmentTitle.add(title);
@NonNull
@Override
public Fragment getItem(int position) {
return fragments.get(position);
@Override
public int getCount() {
return fragments.size();
@Nullable
@Override
public CharSequence getPageTitle(int position) {
return fragmentTitle.get(position);
Step 5: Working with the MainActivity.java file
In the MainActivity, we need to perform the following steps.
- Initialize the ViewPager, TabLayout, and the Adapter.
- Add the Pages (fragments ) along with the titles
- Link the TabLayout to the Viewpager using the setupWithiewPager method.
Syntax:TabLayout.setupWithiewPager(ViewPager pager).
Description: The tablayout with the viewpager. The titles of each pager now appears on the tablayout. The user can also navigate through the fragments by clicking on the tabs.
Parameter:
Viewpager: Used to display the fragments.
Below is the code for the MainActivity.java file. Comments are added inside the code to understand the code in more detail.
import android.os.Bundle;
import androidx.appcompat.app.AppCompatActivity;
import androidx.viewpager.widget.ViewPager;
import com.google.android.material.tabs.TabLayout;
public class MainActivity extends AppCompatActivity {
private ViewPagerAdapter viewPagerAdapter;
private ViewPager viewPager;
private TabLayout tabLayout;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
viewPager = findViewById(R.id.viewpager);
// setting up the adapter
viewPagerAdapter = new ViewPagerAdapter(getSupportFragmentManager());
// add the fragments
viewPagerAdapter.add(new Page1(), "Page 1");
viewPagerAdapter.add(new Page2(), "Page 2");
viewPagerAdapter.add(new Page3(), "Page 3");
// Set the adapter
viewPager.setAdapter(viewPagerAdapter);
// The Page (fragment) titles will be displayed in the
// tabLayout hence we need to set the page viewer
// we use the setupWithViewPager().
tabLayout = findViewById(R.id.tab_layout);
tabLayout.setupWithViewPager(viewPager);
Output:
Find the code in the following GitHub repo: https://github.com/evilc3/ViewPager
Software Setup and Configuration
-
Download and Install Java Development Kit (JDK) on Windows, Mac, and Linux
Setting up a Suitable Development Environment is necessary before one can begin creating any applications. It makes it easier for developers to use the tools required to create any Application and ensures that all Operations or Processes run smoothly. Download and Install JDK in order to create Andr
6 min read
Guide to Install and Setup IntelliJ IDEA for Android App Development
To start developing Android applications, one has to set up a proper development environment. It facilitates developers to use the tools needed in creating an app and ensure that all operations/processes carried out in a smooth manner. An IDE(Integrated Development Environment) is a complete applica
5 min read
Guide to Install and Setup Visual Studio for Android App Development
To start developing Android applications, one has to set up a proper development environment. It facilitates developers to use the tools needed in creating an app and ensure that all operations/processes carried out in a smooth manner. An IDE(Integrated Development Environment) is a complete applica
4 min read
How to Run the Android App on a Real Device?
The time comes when the Android Studio project is ready and you want to test that application. One can test the application by running the application which can be done in two ways. By running the app on an Android Virtual Device(AVD), andBy running the app on a real device So in this article, we ar
2 min read
File Structure & Components
-
Components of an Android Application
There are some necessary building blocks that an Android application consists of. These loosely coupled components are bound by the application manifest file which contains the description of each component and how they interact. The manifest file also contains the app’s metadata, its hardware confi
4 min read
Core Topics
-
How Does Android App Work?
Developing an android application involves several processes that happen in a sequential manner. After writing the source code files, when developers click the Run button on the Android studio, plenty of operations and process starts at the backend. Every operation happening in the background is a c
7 min read
Activity Lifecycle in Android with Demo App
In Android, an activity is referred to as one screen in an application. It is very similar to a single window of any desktop application. An Android app consists of one or more screens or activities. Each activity goes through various stages or a lifecycle and is managed by activity stacks. So when
10 min read
Introduction to Gradle
Gradle is an excellent open-source construction tool that is capable of the development of any kind of software. This tool was developed by a gaggle of developers named Hans Dockter, Szczepan Faber Adam Murdoch, Luke Daley, Peter Niederwieser, Daz DeBoer, and Rene Gröschkebefore 13 years before. It
8 min read
What is Context in Android?
Android Applications are popular for a long time and it is evolving to a greater level as users' expectations are that they need to view the data that they want in an easier smoother view. Hence, the android developers must know the important terminologies before developing the app. In Android Progr
9 min read
Bundle in Android with Example
It is known that Intents are used in Android to pass to the data from one activity to another. But there is one another way, that can be used to pass the data from one activity to another in a better way and less code space ie by using Bundles in Android. Android Bundles are generally used for passi
7 min read
Layout & View
-
Layouts in Android UI Design
Layout Managers (or simply layouts) are said to be extensions of the ViewGroup class. They are used to set the position of child Views within the UI we are building. We can nest the layouts, and therefore we can create arbitrarily complex UIs using a combination of layouts. There is a number of layo
2 min read
Android UI Layouts
Layouts in Android is used to define the user interface that holds the UI controls or widgets that will appear on the screen of an android application or activity screen. Generally, every application is a combination of View and ViewGroup. As we know, an android application contains a large number o
5 min read
LinearLayout and its Important Attributes with Examples in Android
LinearLayout is the most basic layout in android studio, that aligns all the children sequentially either in a horizontal manner or a vertical manner by specifying the android:orientation attribute. If one applies android:orientation="vertical" then elements will be arranged one after another in a v
3 min read
Android LinearLayout in Kotlin
Android LinearLayout is a ViewGroup subclass, used to provide child View elements one by one either in a particular direction either horizontally or vertically based on the orientation property. We can specify the linear layout orientation using the android:orientation attribute. All the child eleme
2 min read
Android RelativeLayout in Kotlin
Android RelativeLayout is a ViewGroup subclass, used to specify the position of child View elements relative to each other like (A to the right of B) or relative to the parent (fixed to the top of the parent). Instead of using LinearLayout, we have to use RelativeLayout to design the user interface
4 min read
Button
-
Button in Android
In Android applications, a Button is a user interface that is used to perform some action when clicked or tapped. It is a very common widget in Android and developers often use it. This article demonstrates how to create a button in Android Studio. The Class Hierarchy of the Button Class in Kotlin X
3 min read
Android | How to add Radio Buttons in an Android Application?
Android radio button is a widget that can have more than one option to choose from. The user can choose only one option at a time. Each option here refers to a radio button and all the options for the topic are together referred to as Radio Group. Hence, Radio Buttons are used inside a RadioGroup. P
6 min read
RadioButton in Kotlin
Android Radio Button is bi-state button which can either be checked or unchecked. Also, it's working is same as Checkbox except that radio button can not allow to be unchecked once it was selected. Generally, we use RadioButton controls to allow users to select one option from multiple options. By d
4 min read
How to add Toggle Button in an Android Application
ToggleButton is basically a stop/play or on/off button with an indicator light indicating the current state of ToggleButton. ToggleButton is widely used, some examples are on/off audio, Bluetooth, WiFi, hot-spot etc. This is a subclass of Composite Button. [video loading="lazy" mp4="https://media.ge
4 min read
Intent and Intent Filters
-
What is Intent in Android?
In Android, it is quite usual for users to witness a jump from one application to another as a part of the whole process, for example, searching for a location on the browser and witnessing a direct jump into Google Maps or receiving payment links in Messages Application (SMS) and on clicking jumpin
4 min read
Implicit and Explicit Intents in Android with Examples
Pre-requisites: Android App Development Fundamentals for Beginners Guide to Install and Set up Android Studio Android | Starting with the first app/android project Android | Running your first Android app This article aims to tell about the Implicit and Explicit intents and how to use them in an and
7 min read
How to Send Data From One Activity to Second Activity in Android?
This article aims to tell and show how to "Send the data from one activity to second activity using Intent" . In this example, we have two activities, activity_first which are the source activity, and activity_second which is the destination activity. We can send the data using the putExtra() method
7 min read
How to open dialer in Android through Intent?
The phone dialer is an activity available with the Android operating system to call a number. Usually, such activity may or may not have an EditText, for taking the number as input, and a Call button. When the user presses the Call button, it invokes the dialer app activity. Use of 'tel:' prefix is
4 min read
Toast & RecyclerView
-
Toasts for Android Studio
A toast provides a simple popup message that is displayed on the current activity UI screen (e.g. Main Activity). Example: Syntax: // to get Context Context context = getApplicationContext(); // message to display String text = "Toast message"; // toast time duration, can also set manual value in
2 min read
What is Toast and How to Use it in Android with Examples?
Pre-requisites: Android App Development Fundamentals for BeginnersGuide to Install and Set up Android StudioAndroid | Starting with the first app/android projectAndroid | Running your first Android appWhat is Toast in Android? A Toast is a feedback message. It takes a very little space for displayin
6 min read
Android Toast in Kotlin
A Toast is a short alert message shown on the Android screen for a short interval of time. Android Toast is a short popup notification which is used to display information when we perform any operation in our app. In this tutorial, we shall not just limit ourselves by creating a lame toast but also
4 min read
Android | How to Change Toast font?
A Toast is a feedback message. It takes a very little space for displaying while overall activity is interactive and visible to the user. It disappears after a few seconds. It disappears automatically. If user wants permanent visible message, Notification can be used. Toast disappears automatically
3 min read
How to add a custom styled Toast in Android
A Toast is a feedback message. It takes very little space for displaying and it is displayed on top of the main content of an activity, and only remains visible for a short time period. This article explains how to create Custom Toast messages, which has custom background, image, icon, etc, which ar
3 min read
Fragments & Adapters
-
Introduction to Fragments | Android
Fragment is a piece of an activity that enables a more modular activity design. A fragment encapsulates functionality so that it is easier to reuse within activities and layouts. Android devices exist in a variety of screen sizes and densities. Fragments simplify the reuse of components in different
5 min read
Fragment Lifecycle in Android
In Android, the fragment is the part of the Activity that represents a portion of the User Interface(UI) on the screen. It is the modular section of the Android activity that is very helpful in creating UI designs that are flexible in nature and auto-adjustable based on the device screen size. The U
8 min read
How to Create a New Fragment in Android Studio?
Android Studio is the official integrated development environment for Google's Android operating system, built on JetBrains' IntelliJ IDEA software and designed specifically for Android development. You can Develop Android App using this. Here, We are going to learn how to create a new Fragment in A
2 min read
How to Create Swipe Navigation in Android?
When talking about Android Apps, the first thing that comes to mind is variety. There are so many varieties of Android apps providing the user with a beautiful dynamic UI. One such feature is to navigate our Android Apps using left and right swipes as opposed to clicking on buttons. Not only does it
6 min read
ViewPager Using Fragments in Android with Example
ViewPager is a layout manager that allows the user to flip left and right through pages of data. It is mostly found in apps like Youtube, Snapchat where the user shifts right - left to switch to a screen. Instead of using activities fragments are used. It is also used to guide the user through the a
7 min read
UI Component & Image Loading Libraries
-
Spinner in Android with Example
Android Spinner is a view similar to the dropdown list which is used to select one option from the list of options. It provides an easy way to select one item from the list of items and it shows a dropdown list of all values when we click on it. The default value of the android spinner will be the c
4 min read
Spinner in Kotlin
Android Spinner is a view similar to a dropdown list which is used to select one option from the list of options. It provides an easy way to select one item from the list of items and it shows a dropdown list of all values when we click on it. The default value of the android spinner will be the cur
3 min read
Dynamic Spinner in Kotlin
Android Spinner is a view similar to dropdown list which is used to select one option from the list of options. It provides an easy way to select one item from the list of items and it shows a dropdown list of all values when we click on it. Default value of the android spinner will be currently sel
3 min read
How to add Custom Spinner in android?
Spinner is a widget that is used to select an item from a list of items. When the user tap on a spinner a drop-down menu is visible to the user. In this article, we will learn how to add custom spinner in the app. If you want to know more about spinner in detail then click on this link. Approach: Cr
4 min read
How to Create an Alert Dialog Box in Android?
Alert Dialog shows the Alert message and gives the answer in the form of yes or no. Alert Dialog displays the message to warn you and then according to your response, the next step is processed. Android Alert Dialog is built with the use of three fields: Title, Message area, and Action Button. Alert
4 min read
Date and Time
-
DatePicker in Kotlin
Android DatePicker is a user interface control which is used to select the date by day, month and year in our android application. DatePicker is used to ensure that the users will select a valid date.In android DatePicker having two modes, first one to show the complete calendar and second one shows
3 min read
TimePicker in Kotlin
Android TimePicker is a user interface control for selecting the time in either 24-hour format or AM/PM mode. It is used to ensure that users pick the valid time for the day in our application. In android, TimePicker is available in two modes first one is clock mode and another one is spinner mode.
4 min read
Android | How to display Analog clock and Digital clock
Pre-requisites: Android App Development Fundamentals for Beginners Guide to Install and Set up Android Studio Android | Starting with first app/android project Android | Running your first Android app Analog and digital clocks are used for display the time in android application. Analog clock: Analo
2 min read
Android | Creating a Calendar View app
This article shows how to create an android application for displaying the Calendar using CalendarView. It also provides the selection of the current date and displaying the date. The setOnDateChangeListener Interface is used which provide onSelectedDayChange method. onSelectedDayChange: In this met
3 min read
PulseCountDown in Android with Example
PulseCountDown in Android is an alternative to CountDownTimer. A CountDownTimer is an accurate timer that can be used for a website or blog to display the count-down to any special event, such as a birthday or anniversary. It is very easy to implement PulseCountDown instead of CountDownTimer because
3 min read
Material Design & Bars
-
Introduction to Material Design in Android
Material Design Components (MDC Android) offers designers and developers a way to implement Material Design in their Android application. Developed by a core team of engineers and UX designers at Google, these components enable a reliable development workflow to build beautiful and functional Androi
5 min read
Responsive UI Design in Android
Nowadays every Android UI designer is very serious about his UI design. The path of every project goes through UI design. UI design is the face of every project and every website, app & desktop application. UI is the wall between the User & Back-end where users can interact with the server t
4 min read
Material Design EditText in Android with Example
EditText is one of the important UI elements. Edittext refers to the widget that displays an empty text field in which a user can enter the required text and this text is further used inside the application. In this article it has been discussed to implement the special type of text fields, those ar
5 min read
Theming of Material Design EditText in Android with Example
Prerequisite: Material Design EditText in Android with Examples In the previous article Material Design EditText in Android with Examples Material design Text Fields offers more customization and makes the user experience better than the normal text fields. For example, Icon to clear the entire Edit
4 min read
Key Properties of Material Design EditText in Android
In the previous article Material Design EditText in Android with Examples its been discussed how to implement the Material design EditText and how they differ from the normal EditText. In this article its been discussed on how to customize MDC edit text fields with their key properties. Have a look
4 min read
Working with Google Maps
-
How to Generate API Key for Using Google Maps in Android?
For using any Google services in Android, we have to generate an API key or we have to generate some unique key for using that service provided by Google. So Maps is one of the famous and most used services provided by Google. Maps can be seen in each and every app provided by Google and we also can
3 min read
How to Add Custom Marker to Google Maps in Android?
Google Maps is used in many apps for displaying a location and indicating a specific location on a map. We have seen the use of maps in many apps that provides services such as Ola, Uber, and many more. In these apps, you will get to see How we can add Custom Marker to Google Maps in Android. What w
5 min read
How to Add Multiple Markers on Google Maps in Android?
Google Map is used in most of the apps which are used to represent many locations and markers on Google Maps. We have seen markers on Google maps for multiple locations. In this article, we will take a look at the implementation of Multiple Markers on Google Maps in Android. What we are going to bui
4 min read
How to Use Different Types of Google Maps in Android?
When we use the default application of Google Maps we will get to see different types of Maps present inside this application. We will get to see satellite maps, terrain maps, and many more. We have seen adding Google Maps in the Android application. In this article, we will take a look at the imple
4 min read
How to Add SearchView in Google Maps in Android?
We have seen the implementation of Google Maps in Android along with markers on it. But many apps provide features so that users can specify the location on which they have to place markers. So in this article, we will implement a SearchView in our Android app so that we can search a location name a
4 min read
Chart & Animation
-
How to add a Pie Chart into an Android Application
A Pie Chart is a circular statistical graphic, which is divided into slices to illustrate numerical proportions. It depicts a special chart that uses “pie slices”, where each sector shows the relative sizes of data. A circular chart cuts in the form of radii into segments describing relative frequen
7 min read
Point Graph Series in Android
We have seen using a simple line graph and BarChart implementation in Android to represent data in the graphical format. Another graphical format for representing data is a point graph series. In this article, we will take a look at the implementation of the Point Graph Series in Android. What we ar
3 min read
How to Create Group BarChart in Android?
As we have seen how we can create a beautiful bar chart in Android but what if we have to represent data in the form of groups in our bar chart. So that we can plot a group of data in our bar chart. So we will be creating a Group Bar Chart in our Android app in this article. What we are going to bui
5 min read
How to Create a BarChart in Android?
If you are looking for a UI component to represent a huge form of data in easily readable formats then you can think of displaying this huge data in the form of bar charts or bar graphs. It makes it easy to analyze and read the data with the help of bar graphs. In this article, we will take a look a
4 min read
Line Graph View in Android with Example
If you are looking for a view to represent some statistical data or looking for a UI for displaying a graph in your app then in this article we will take a look on creating a line graph view in our Android App using the GraphView library. What we are going to build in this article? We will be build
3 min read
Database
-
Firebase - Introduction
Firebase is a product of Google which helps developers to build, manage, and grow their apps easily. It helps developers to build their apps faster and in a more secure way. No programming is required on the firebase side which makes it easy to use its features more efficiently. It provides services
5 min read
Adding Firebase to Android App
There are various services offered online such as storage, online processing, realtime database, authorisation of user etc. Google developed a platform called Firebase that provide all these online services. It also gives a daily analysis of usage of these services along with the details of user usi
3 min read
How to use Firebase UI Authentication Library in Android?
Firebase UI is a library provided by Firebase for Android apps which makes or so many tasks easy while integrating Firebase in Android. This library provides so many extra features that we can integrate into our Android very easily. In this article, we will take a look at using this library for addi
10 min read
User authentication using Firebase in Android
Firebase is a mobile and web application development platform. It provides services that a web application or mobile application might require. Firebase provides email and password authentication without any overhead of building backend for user authentication. Steps for firebase user authentication
7 min read
Firebase Authentication with Phone Number OTP in Android
Many apps require their users to be authenticated. So for the purpose of authenticating the apps uses phone number authentication inside their apps. In phone authentication, the user has to verify his identity with his phone number. Inside the app user has to enter his phone number after that he wil
9 min read
Advance Android
-
Shared Preferences in Android with Example
One of the most Interesting Data Storage options Android provides its users is Shared Preferences. Shared Preferences is the way in which one can store and retrieve small amounts of primitive data as key/value pairs to a file on the device storage such as String, int, float, Boolean that make up you
8 min read
Internal Storage in Android with Example
The aim of this article is to show users how to use internal storage. In this article will be creating an application that can write data to a file and store it in internal storage and read data from the file and display it on the main activity using TextView. Saving and loading data on the internal
5 min read
External Storage in Android with Example
Android gives various options for storing apps data which uses a file system similar to the disk-based system on computer platforms App-Specific storage: Store data files within internal volume directories or external. These data files are meant only for the app's use. It uses internal storage direc
9 min read
How to Save ArrayList to SharedPreferences in Android?
SharedPreferences in Android is local storage that is used to store strings, integers, and variables in phone storage so that we can manage the state of the app. We have seen storing simple variables in shared prefs with key and value pair. In this article, we will see How we can store ArrayList to
7 min read
Preferences DataStore in Android
Preference Data Store is used to store data permanently in android. Earlier we had to Shared Preferences for the same but since it is deprecated we are using Data Store now. A sample video is given below to get an idea about what we are going to do in this article. Note that we are going to implemen
4 min read
Jetpack & Architecture
-
Introduction to Android Jetpack
The Android support libraries are used in almost all android applications to overcome the compatibility issues across different Android OS versions and devices. These libraries also facilitate users to add various kinds of updated widgets in the application. Over time, these libraries are updated ac
6 min read
Foundation Components of Android Jetpack
Android Jetpack is a set of software components, libraries, tools, and guidance to help in developing robust Android applications. Launched by Google in 2018, Jetpack comprises existing android support libraries, android architecture components with an addition of the Android KTX library as a single
7 min read
Jetpack Architecture Components in Android
Android Jetpack is a set of software components, libraries, tools, and guidance to help in developing robust Android applications. Launched by Google in 2018, Jetpack comprises existing android support libraries, android architecture components with an addition of the Android KTX library as a single
10 min read
Behaviour Components of Android Jetpack
Android Jetpack is a set of software components, libraries, tools, and guidance to help in developing robust Android applications. Launched by Google in 2018, Jetpack comprises existing android support libraries, android architecture components with an addition of the Android KTX library as a single
10 min read
UI Components of Android Jetpack
Android Jetpack is a set of software components, libraries, tools, and guidance to help in developing robust Android applications. Launched by Google in 2018, Jetpack comprises existing android support libraries, android architecture components with an addition of the Android KTX library as a single
14 min read
App Publish & App Monetization
-
How to Publish Your Android App on Google Play Store?
Nowadays smartphones are among the most essential gadgets for users. Over 60% of people sleep with their phones by their side and check it first thing in the morning. Almost all businesses, including retail stores, have mobile apps to keep their audience engaged. Various applications like games, mus
5 min read
How to Publish Your Android App on Amazon App Store for Free?
To publish your android application on the play store, you need to pay some particular amount to Google, and only then google will let you publish your application. Here, the big question is, what if you don't have money to publish your application? what will you do in that situation? The best solut
3 min read
Overview of Google Admob
In order to earn money from the Android app or game, there are several ways such as in-App Purchases, Sponsorship, Advertisements, and many more. But there is another conventional approach to earn money from the Android app is by integrating an advertisement which is known as Google AdMob. Google Ad
4 min read
Android | AdMob Banner Ads for Android Studio
Banner ads are a rectangular image or text ads that occupy a spot within an app's layout. If you're new to mobile advertising, banner ads are the easiest to implement. This article shows you how to integrate banner ads from AdMob into an Android app.Example: First, create a new project in Android St
2 min read
Android | AdMob Interstitial Ads for Android Studio
Interstitial ads are full-screen ads that cover the whole UI of the app. This article shows you how to integrate Interstitial ads from AdMob into an Android app. Example - First Create a new Project in Android Studio and add the following codes to import the google Mobile Ads SDK. In the project-lev
2 min read
Projects
-
How to Build a Tic Tac Toe Game in Android?
In this article, we will be building a Tic Tac Toe Game Project using Java and XML in Android. The Tic Tac Toe Game is based on a two-player game. Each player chooses between X and O. The Player plays one move at a time simultaneously. In a move, a player can choose any position from a 3x3 grid. The
9 min read
How to create a Face Detection Android App using Machine Learning KIT on Firebase
Pre-requisites: Firebase Machine Learning kitAdding Firebase to Android AppFirebase ML KIT aims to make machine learning more accessible, by providing a range of pre-trained models that can use in the iOS and Android apps. Let's use ML Kit’s Face Detection API which will identify faces in photos. By
9 min read
How to Build a Simple Augmented Reality Android App?
Augmented Reality has crossed a long way from Sci-fi Stories to Scientific reality. With this speed of technical advancement, it's probably not very far when we can also manipulate digital data in this real physical world as Tony Stark did in his lab. When we superimpose information like sound, text
11 min read
How to Build a Grocery Android App using MVVM and Room Database?
In this article, we are going to build a grocery application in android using android studio. Many times we forget to purchase things that we want to buy, after all, we can’t remember all the items, so with the help of this app, you can note down your grocery items that you are going to purchase, by
15+ min read
Android Projects - From Basic to Advanced Level
Android, built on a modified version of the Linux kernel, is the go-to operating system for touchscreen mobile devices like smartphones and tablets. For anyone aspiring to become an Android developer, diving into Android projects is essential. Engaging in a variety of projects, from simple apps to c
11 min read
Top 25 Android Interview Questions and Answers For Experienced
The need for new technologies and their developers is increasing as the world is dynamically transforming digitally. Everyone nowadays relies on apps for a variety of functions, including acquiring information and keeping in touch with one another as well as daily activities like shopping, commuting
15+ min read
Top 50 Android Interview Questions and Answers - SDE I to SDE III
A Linux-based open-source OS, Android was created by Andy Rubin and became one of the most popular smartphone operating systems. With 71 percent of the market share worldwide, Android is on top. Because it is on top in the smartphone OS, Android development is always in demand. If you are seeking a
15+ min read
- Company
- About Us
- Legal
- In Media
- Contact Us
- Advertise with us
- GFG Corporate Solution
- Placement Training Program
- GeeksforGeeks Community
- DSA
- Data Structures
- Algorithms
- DSA for Beginners
- Basic DSA Problems
- DSA Roadmap
- Top 100 DSA Interview Problems
- DSA Roadmap by Sandeep Jain
- All Cheat Sheets
- Computer Science
- Operating Systems
- Computer Network
- Database Management System
- Software Engineering
- Digital Logic Design
- Engineering Maths
- Software Development
- Software Testing
- System Design
- High Level Design
- Low Level Design
- UML Diagrams
- Interview Guide
- Design Patterns
- OOAD
- System Design Bootcamp
- Interview Questions
We use cookies to ensure you have the best browsing experience on our website. By using our site, you
acknowledge that you have read and understood our
Cookie Policy &
Privacy Policy
Cookies are not collected in the GeeksforGeeks mobile applications.
Got It !
![]() |
悲伤的山羊 · 蓝牙版T8FB常见问题 5 月前 |